daily leetcode - remove-duplicates-from-sorted-array-ii - !
题目地址
https://leetcode.com/problems/remove-duplicates-from-sorted-array-ii/
题目描述
Given a sorted array nums , remove the duplicates in-place such that duplicates appeared at most twice and return the new length.
Do not allocate extra space for another array, you must do this by modifying the input array in-place with O(1) extra memory.
Example 1:
Given _nums_ = [1,1,1,2,2,3],
Your function should return length = 5, with the first five elements of _nums_ being 1, 1, 2, 2 and 3 respectively.
It doesn't matter what you leave beyond the returned length.
Example 2:
Given _nums_ = [0,0,1,1,1,1,2,3,3],
Your function should return length = 7, with the first seven elements of _nums_ being modified to 0, 0, 1, 1, 2, 3 and 3 respectively.
It doesn't matter what values are set beyond the returned length.
Clarification:
Confused why the returned value is an integer but your answer is an array?
Note that the input array is passed in by reference, which means modification to the input array will be known to the caller as well.
Internally you can think of this:
// nums is passed in by reference. (i.e., without making a copy)
int len = removeDuplicates(nums);
// any modification to nums in your function would be known by the caller.
// using the length returned by your function, it prints the first len elements.
for (int i = 0; i < len; i++) {
print(nums[i]);
}
思路
这道题是之前那道 Remove Duplicates from Sorted Array 的拓展,这里允许最多重复的次数是两次,那么可以用一个变量 cnt 来记录还允许有几次重复,cnt 初始化为1,如果出现过一次重复,则 cnt 递减1,那么下次再出现重复,快指针直接前进一步,如果这时候不是重复的,则 cnt 恢复1,由于整个数组是有序的,所以一旦出现不重复的数,则一定比这个数大,此数之后不会再有重复项。理清了上面的思路,则代码很好写了:
解法一:
class Solution {
public:
int removeDuplicates(vector<int>& nums) {
int pre = 0, cur = 1, cnt = 1, n = nums.size();
while (cur < n) {
if (nums[pre] == nums[cur] && cnt == 0) ++cur;
else {
if (nums[pre] == nums[cur]) --cnt;
else cnt = 1;
nums[++pre] = nums[cur++];
}
}
return nums.empty() ? 0 : pre + 1;
}
};
这里其实也可以用类似于 Remove Duplicates from Sorted Array 中的解法三的模版,由于这里最多允许两次重复,那么当前的数字 num 只要跟上上个覆盖位置的数字 nusm[i-2] 比较,若 num 较大,则绝不会出现第三个重复数字(前提是数组是有序的),这样的话根本不需要管 nums[i-1] 是否重复,只要将重复个数控制在2个以内就可以了,参见代码如下:
解法二:
class Solution {
public:
int removeDuplicates(vector<int>& nums) {
int i = 0;
for (int num : nums) {
if (i < 2 || num > nums[i - 2]) {
nums[i++] = num;
}
}
return i;
}
};
思路2
”删除排序“类题目截止到现在(2020-1-15)一共有四道题:
这道题是26.remove-duplicates-from-sorted-array 的进阶版本,唯一的不同是不再是全部元素唯一,而是全部元素不超过 2 次。实际上这种问题可以更抽象一步,即“删除排序数组中的重复项,使得相同数字最多出现 k 次”
。 那么这道题 k 就是 2, 26.remove-duplicates-from-sorted-array 的 k 就是 1。
上一题我们使用了快慢指针来实现,这道题也是一样,只不过逻辑稍有不同。 其实快慢指针本质是读写指针,在这里我们的快指针实际上就是读指针,而慢指针恰好相当于写指针。”快慢指针的说法“便于描述和记忆,“读写指针”的说法更便于理解本质。本文中,以下内容均描述为快慢指针。
- 初始化快慢指针 slow , fast ,全部指向索引为 0 的元素。
- fast 每次移动一格
- 慢指针选择性移动,即只有写入数据之后才移动。是否写入数据取决于 slow - 2 对应的数字和 fast 对应的数字是否一致。
- 如果一致,我们不应该写。 否则我们就得到了三个相同的数字,不符合题意
- 如果不一致,我们需要将 fast 指针的数据写入到 slow 指针。
- 重复这个过程,直到 fast 走到头,说明我们已无数字可写。
图解(红色的两个数字,表示我们需要比较的两个数字):
关键点解析
- 快慢指针
- 读写指针
- 删除排序问题
代码
代码支持: Python
Python Code:
class Solution:
def removeDuplicates(self, nums: List[int]) -> int:
# 写指针
i = 0
K = 2
for num in nums:
if i < K or num != nums[i-K]:
nums[i] = num
i += 1
return i
基于这套代码,你可以轻易地实现 k 为任意正整数的算法。
相关题目
正如上面所说,相关题目一共有三道(排除自己)。其中一道我们仓库已经讲到了。剩下两道原理类似,但是实际代码和细节有很大不同,原因就在于数组可以随机访问,而链表不行。 感兴趣的可以做一下剩下的两道链表题。
-
- 删除排序链表中的重复元素 II
-
- 删除排序链表中的重复元素
本文参考自:
https://github.com/grandyang/leetcode/ &
https://github.com/azl397985856/leetcode
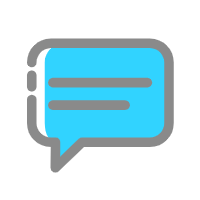