daily leetcode - reverse-nodes-in-k-group - !
题目地址
https://leetcode.com/problems/reverse-nodes-in-k-group/
题目描述
Given a linked list, reverse the nodes of a linked list k at a time and return its modified list.
k is a positive integer and is less than or equal to the length of the linked list. If the number of nodes is not a multiple of k then left-out nodes in the end should remain as it is.
Example:
Given this linked list: 1->2->3->4->5
For k = 2, you should return: 2->1->4->3->5
For k = 3, you should return: 3->2->1->4->5
Note:
- Only constant extra memory is allowed.
- You may not alter the values in the list's nodes, only nodes itself may be changed.
思路
这道题让我们以每k个为一组来翻转链表,实际上是把原链表分成若干小段,然后分别对其进行翻转,那么肯定总共需要两个函数,一个是用来分段的,一个是用来翻转的,以题目中给的例子来看,对于给定链表 1->2->3->4->5,一般在处理链表问题时,大多时候都会在开头再加一个 dummy node,因为翻转链表时头结点可能会变化,为了记录当前最新的头结点的位置而引入的 dummy node,加入 dummy node 后的链表变为 -1->1->2->3->4->5,如果k为3的话,目标是将 1,2,3 翻转一下,那么需要一些指针,pre 和 next 分别指向要翻转的链表的前后的位置,然后翻转后 pre 的位置更新到如下新的位置:
-1->1->2->3->4->5
| | |
pre cur next
-1->3->2->1->4->5
| | |
cur pre next
以此类推,只要 cur 走过k个节点,那么 next 就是 cur->next,就可以调用翻转函数来进行局部翻转了,注意翻转之后新的 cur 和 pre 的位置都不同了,那么翻转之后,cur 应该更新为 pre->next,而如果不需要翻转的话,cur 更新为 cur->next,代码如下所示:
解法一:
class Solution {
public:
ListNode* reverseKGroup(ListNode* head, int k) {
if (!head || k == 1) return head;
ListNode *dummy = new ListNode(-1), *pre = dummy, *cur = head;
dummy->next = head;
for (int i = 1; cur; ++i) {
if (i % k == 0) {
pre = reverseOneGroup(pre, cur->next);
cur = pre->next;
} else {
cur = cur->next;
}
}
return dummy->next;
}
ListNode* reverseOneGroup(ListNode* pre, ListNode* next) {
ListNode *last = pre->next, *cur = last->next;
while(cur != next) {
last->next = cur->next;
cur->next = pre->next;
pre->next = cur;
cur = last->next;
}
return last;
}
};
我们也可以在一个函数中完成,首先遍历整个链表,统计出链表的长度,然后如果长度大于等于k,交换节点,当 k=2 时,每段只需要交换一次,当 k=3 时,每段需要交换2此,所以i从1开始循环,注意交换一段后更新 pre 指针,然后 num 自减k,直到 num<k 时循环结束,参见代码如下:
解法二:
class Solution {
public:
ListNode* reverseKGroup(ListNode* head, int k) {
ListNode *dummy = new ListNode(-1), *pre = dummy, *cur = pre;
dummy->next = head;
int num = 0;
while (cur = cur->next) ++num;
while (num >= k) {
cur = pre->next;
for (int i = 1; i < k; ++i) {
ListNode *t = cur->next;
cur->next = t->next;
t->next = pre->next;
pre->next = t;
}
pre = cur;
num -= k;
}
return dummy->next;
}
};
我们也可以使用递归来做,用 head 记录每段的开始位置,cur 记录结束位置的下一个节点,然后调用 reverse 函数来将这段翻转,然后得到一个 new_head,原来的 head 就变成了末尾,这时候后面接上递归调用下一段得到的新节点,返回 new_head 即可,参见代码如下:
解法三:
class Solution {
public:
ListNode* reverseKGroup(ListNode* head, int k) {
ListNode *cur = head;
for (int i = 0; i < k; ++i) {
if (!cur) return head;
cur = cur->next;
}
ListNode *new_head = reverse(head, cur);
head->next = reverseKGroup(cur, k);
return new_head;
}
ListNode* reverse(ListNode* head, ListNode* tail) {
ListNode *pre = tail;
while (head != tail) {
ListNode *t = head->next;
head->next = pre;
pre = head;
head = t;
}
return pre;
}
};
思路2
题意是以 k
个nodes为一组进行翻转,返回翻转后的linked list
.
从左往右扫描一遍linked list
,扫描过程中,以k为单位把数组分成若干段,对每一段进行翻转。给定首尾nodes,如何对链表进行翻转。
链表的翻转过程,初始化一个为null
的 previous node(prev)
,然后遍历链表的同时,当前node (curr)
的下一个(next)指向前一个node(prev)
,
在改变当前node的指向之前,用一个临时变量记录当前node的下一个node(curr.next)
. 即
ListNode temp = curr.next;
curr.next = prev;
prev = curr;
curr = temp;
举例如图:翻转整个链表 1->2->3->4->null
-> 4->3->2->1->null
这里是对每一组(k个nodes
)进行翻转,
-
先分组,用一个
count
变量记录当前节点的个数 -
用一个
start
变量记录当前分组的起始节点位置的前一个节点 -
用一个
end
变量记录要翻转的最后一个节点位置 -
翻转一组(
k个nodes
)即(start, end) - start and end exclusively
。 -
翻转后,
start
指向翻转后链表, 区间(start,end)
中的最后一个节点, 返回start
节点。 -
如果不需要翻转,
end
就往后移动一个(end=end.next
),每一次移动,都要count+1
.
如图所示 步骤4和5: 翻转区间链表区间(start, end)
举例如图,head=[1,2,3,4,5,6,7,8], k = 3
NOTE: 一般情况下对链表的操作,都有可能会引入一个新的
dummy node
,因为head
有可能会改变。这里head 从1->3
,
dummy (List(0))
保持不变。
复杂度分析
- 时间复杂度:
O(n) - n is number of Linked List
- 空间复杂度:
O(1)
关键点解析
- 创建一个dummy node
- 对链表以k为单位进行分组,记录每一组的起始和最后节点位置
- 对每一组进行翻转,更换起始和最后的位置
- 返回
dummy.next
.
代码
Java Code
class ReverseKGroupsLinkedList {
public ListNode reverseKGroup(ListNode head, int k) {
if (head == null || k == 1) {
return head;
}
ListNode dummy = new ListNode(0);
dummy.next = head;
ListNode start = dummy;
ListNode end = head;
int count = 0;
while (end != null) {
count++;
// group
if (count % k == 0) {
// reverse linked list (start, end]
start = reverse(start, end.next);
end = start.next;
} else {
end = end.next;
}
}
return dummy.next;
}
/**
* reverse linked list from range (start, end), return last node.
* for example:
* 0->1->2->3->4->5->6->7->8
* | |
* start end
*
* After call start = reverse(start, end)
*
* 0->3->2->1->4->5->6->7->8
* | |
* start end
* first
*
*/
private ListNode reverse(ListNode start, ListNode end) {
ListNode curr = start.next;
ListNode prev = start;
ListNode first = curr;
while (curr != end){
ListNode temp = curr.next;
curr.next = prev;
prev = curr;
curr = temp;
}
start.next = prev;
first.next = curr;
return first;
}
}
Python3 Cose
class Solution:
def reverseKGroup(self, head: ListNode, k: int) -> ListNode:
if head is None or k < 2:
return head
dummy = ListNode(0)
dummy.next = head
start = dummy
end = head
count = 0
while end:
count += 1
if count % k == 0:
start = self.reverse(start, end.next)
end = start.next
else:
end = end.next
return dummy.next
def reverse(self, start, end):
prev, curr = start, start.next
first = curr
while curr != end:
temp = curr.next
curr.next = prev
prev = curr
curr = temp
start.next = prev
first.next = curr
return first
参考(References)
扩展
-
要求从后往前以
k
个为一组进行翻转。(字节跳动(ByteDance)面试题)例子,
1->2->3->4->5->6->7->8, k = 3
,从后往前以
k=3
为一组,6->7->8
为一组翻转为8->7->6
,3->4->5
为一组翻转为5->4->3
.1->2
只有2个nodes少于k=3
个,不翻转。
最后返回:
1->2->5->4->3->8->7->6
这里的思路跟从前往后以k
个为一组进行翻转类似,可以进行预处理:
-
翻转链表
-
对翻转后的链表进行从前往后以k为一组翻转。
-
翻转步骤2中得到的链表。
例子:1->2->3->4->5->6->7->8, k = 3
-
翻转链表得到:
8->7->6->5->4->3->2->1
-
以k为一组翻转:
6->7->8->3->4->5->2->1
-
翻转步骤#2链表:
1->2->5->4->3->8->7->6
本文参考自:
https://github.com/grandyang/leetcode/ &
https://github.com/azl397985856/leetcode
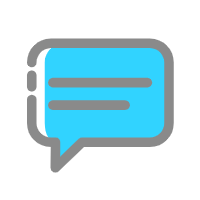